Write to the Event Log in .NET (VB.NET and C#.NET)
Aug 24, 2004 • Chris Pietschmann • Visual Basic • .NETIt is as simple as this to write to the Windows Event Log in .NET
Declaration
Imports System.Diagnostics
Code
Public Function WriteToEventLog(ByVal Entry As String, _
Optional ByVal AppName As String = "APlusFeeCalc", _
Optional ByVal EventType As EventLogEntryType = EventLogEntryType.Information, _
Optional ByVal LogName As String = "Application") As Boolean
Dim objEventLog As New EventLog
Try
'Register the App as an Event Source
If Not objEventLog.SourceExists(AppName) Then
objEventLog.CreateEventSource(AppName, LogName)
End If
objEventLog.Source = AppName
'WriteEntry is overloaded; this is one of 10 ways to call it
objEventLog.WriteEntry(Entry, EventType)
Return True
Catch Ex As Exception
Return False
End Try
End Function
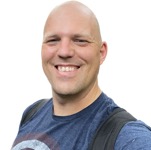
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.