C#: Give your object a Default Indexer Property
Mar 17, 2007 • Chris Pietschmann • C# • .NETI’ve seen/used objects within the .NET Framework that have default indexer properties (ie: SqlDataReader, System.Collections.Generic.List
). Now how exactly do I give my own custom object type a default indexer property?
Well, it’s actually rather simple.
Heres an example of a Club
object with a People
collection of type Person
that has a default indexer property set up:
public class Club
{
// Collection of Person objects in the Club
private List<Person> _people = new List<Person>();
public List<Person> People
{
get { return _people; }
set { _people = value; }
}
// The Default property of the Club object
public Person this[int index]
{
get { return _people[index]; }
set { _people[index] = value; }
}
}
public class Person
{
public Person(string name)
{
this._name = name;
}
private string _name;
public string Name
{
get { return _name; }
set { _name = value; }
}
}
And, here’s example code of using that object:
// Create instance of Club object
Club club = new Club();
// Add a couple Person objects to the People collection of the Club
club.People.Add(new Person("Chris"));
club.People.Add(new Person("Kate"));
// Change the name of the first Person in the People
// collection using the traditional method
club.People[0].Name = "Joe";
// Change the name of the first Person in the People
// collection using the Club object's Default property
club[0].Name = "John";
And, remember just like any other method/property the Default Indexer Property can be overloaded. For instance, you can define one that accepts an Index as type integer, and also have one that accepts an Index of type string that does a dictionary lookup.
Update 3/23/2007: I have updated this post and changed the title, since there seems to be some confusion as to what I’m describing is a Default Property or Indexer Property. I think I’ll just refer to it as a Default Indexer Property. Sorry for the confusion.
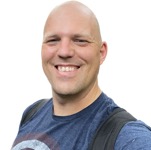
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.