Virtual Earth: Getting Started - Adding a basic Map to a page
Dec 21, 2007 • Chris Pietschmann • MappingWhat is Microsoft Virtual Earth?
Virtual Earth allows any developer to implement mapping technology within their web sites and/or applications. The mapping techology behind Virtual Earth is the exact same technology that powers Microsoft’s Live Maps website.
Getting Started with Virtual Earth
Lets add a Map to a page
Step 1: Add a DOCTYPE declaration and specify UTF-8 as the pages character set at the top of the page
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8">In order for certain map elements to be drawn correctly, you must use UTF-8 encoding.
Step 2: Add a script reference to the JavaScript Virtual Earth Map Control
<script type="text/javascript" src="http://dev.virtualearth.net/mapcontrol/mapcontrol.ashx?v=6"></script>For best results, add this script reference to the page header. Placing this in the header ensures that the script will be loaded before other JavaScript on the page is executed. Doing this will prevent errors occuring because of certain code running before the script file is loaded.
Step 3: Add a DIV to the page where we want the Map to be rendered
This is essentially a placeholder where we will tell Virtual Earth to render the map.
<div id="myMap" style="position:relative; width:400px; height:400px;"></div>You should also specify the Height and Width of the map using CSS styles on the DIV element. If you do not specify a height and width, then Virtual Earth will us the defaults of: 600px width and 400px height.
In this example we are also adding a black border around the map.
Step 4: Tell Virtual Earth to render the map
To do this we just add the following JavaScript to the page after the maps DIV is declared
<script type="text/javascript"> var map = null; map = new VEMap('myMap'); map.LoadMap(); </script>Step 5: Run the page and see the map
Implementation Tips
**Tip 1: **When calling the LoadMap method, it is better practice to place the code that instantiates the map within a method and then have it called within the pages OnLoad event. To set this up do the following:
<body onload="PageLoad()"> <script type="text/javascript"> function PageLoad() { var map = null; map = new VEMap('myMap'); map.LoadMap(); } </script>**Tip 2: **You can also specify a the location (Lat/Long), zoom level and map styles when calling the LoadMap method.
map.LoadMap(new VELatLong(47.6, -122.33), 10, VEMapStyle.Road);Is there an ASP.NET Virtual Earth server control?
Yes, but it isn’t from Microsoft. The Simplovation Web.Maps.VE component is a reusable ASP.NET AJAX Virtual Earth mapping server control that is created with the goal of abstracting out as much JavaScript as possible when implementing Virtual Earth mapping within ASP.NET. It even allows you to handle most map events (like: OnEndZoom, OnEndPan and OnClick, etc.) all from within server-side code; so you don’t even have to write any JavaScript if you don’t want to.
Complete Code For This Article
You can just save the following code in an .htm file and run it in your browser to see the above code in action without typing it all in yourself.
<!DOCTYPE html PUBLIC “-//W3C//DTD XHTML 1.0 Transitional//EN” “http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd”>
<script type="text/javascript" src="http://dev.virtualearth.net/mapcontrol/mapcontrol.ashx?v=6" mce_src="</script">http://dev.virtualearth.net/mapcontrol/mapcontrol.ashx?v=6"></script>Why did I write this?
You may be wondering why I wrote a basic “Getting Started with Virtual Earth” tutorial when there are already other ones out there? Well, it’s simple, I am working on some more advanced articles and I want to make sure you have a basic understanding before I go into more detail.
Additional Resources
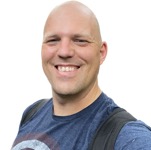
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.