JavaScript int.TryParse Equivalent
Jan 14, 2008 • Chris Pietschmann • JavascriptOne of the most handy methods in .NET is the int.TryParse method. It makes it really convenient when evaluating a string value as an integer. But, JavaScript has no equivalent so you need to do your own evaluation every time.
Here’s a simple JavaScript method I wrote that takes the work out of evaluating a string to an integer:
function TryParseInt(str,defaultValue) {
var retValue = defaultValue;
if(str !== null) {
if(str.length > 0) {
if (!isNaN(str)) {
retValue = parseInt(str);
}
}
}
return retValue;
}

The first parameter of the TryParseInt
method is the string you want to evaluate, and the second parameter is the default value to return if the string cannot be evaluated to an integer.
Here are some example usages:
//This will return 5
var a = TryParseInt("5", 0);
alert(a);
//This will return 0
var b = TryParseInt("5d", 0);
alert(b);
//This will return null
var c = TryParseInt("chris", null);
alert(c);
Happy coding!
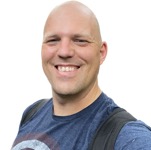
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.