Simple JavaScript Object Reflection API (.NET Style)
Feb 28, 2008 • Chris Pietschmann • JavascriptI was thinking about how JavaScript JSON serializers go about serializing objects. But how does the serializer know about each of the objects properties? I figured JavaScript must have some method of object reflection (similar to .NET Reflection) and it does.
Here’s a simple Reflection namespace that allows you to more easily reflect through an objects methods and properties:
if (typeof PietschSoft == 'undefined') var PietschSoft = {};
if (typeof PietschSoft.Reflection == 'undefined') PietschSoft.Reflection = {};
PietschSoft.Reflection.GetProperties = function(obj){
var props = new Array();
for (var s in obj)
{
if (typeof(obj[s]) != 'function') {
props[props.length] = s;
}
}
return props;
};
PietschSoft.Reflection.GetMethods = function(obj) {
var methods = new Array();
for (var s in obj)
{
if (typeof(obj[s]) == 'function') {
methods[methods.length] = s;
}
}
return methods
};
And, here’s some simple code using the above simple reflection api:
/// Define our Person Object
Person = function() {
this.FirstName = '';
this.LastName = '';
};
Person.prototype.TestFunction = function(){return 'Test Function';};
// Define our instance of the Person object
var p = new Person();
p.FirstName = 'Chris';
p.LastName = 'Pietschmann';
/// Loop through the Objects Properties
var props = PietschSoft.Reflection.GetProperties(p);
for (var i in props)
{
alert(props[i] + ' : ' + p[props[i]]);
}
/// Loop through the Objects Methods
var methods = PietschSoft.Reflection.GetMethods(p);
for (var i in methods)
{
alert(methods[i] + ' : ' + p[methods[i]]);
}
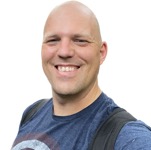
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.