Some ASP.NET AJAX Tips and Tricks
Apr 3, 2008 • Chris Pietschmann • ASP.NETI’ve been using ASP.NET AJAX alot for a while now, so I thought I’d share some of the Tips and Tricks that I’ve learned though my adventures. Lately, all the buzz has been around Silverlight, but I know there are still a lot of devs out there that can benefit from these tips and tricks.
UpdatePanel Tips
Use UpdateMode=Conditional
By setting the UpdatePanel’s UpdateMode property to Conditional, it will only update its contents when one of its triggers occurs or a control within the UpdatePanel itself raises a Postback.
To do this just do the following:
<asp:UpdatePanel runat="server" ID="UpdatePanel_List" UpdateMode="Conditional">
Programmatically Tell the ScriptManager to handle a specific controls postbacks asynchronously
Programmatically telling the ScriptManager to raise a Postbacks Asynchronously can be usefull in some scenarios; such as when you may want to optionally enable ajax for certain things on the page.
To do this you just use the ScriptManagers RegisterAsyncPostBackControl method and pass it the reference to the control you want to make asynchronous.
Here’s an example page that does this:
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="UpdatePanel_01_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>Untitled Page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:ScriptManager runat="server" ID="ScriptManager1"></asp:ScriptManager>
<asp:Button runat="server" ID="btnShowTime" Text="Show Time" OnClick="btnShowTime_Click" />
<asp:UpdatePanel runat="server" ID="UpdatePanel1">
<ContentTemplate>
<asp:Label runat="server" ID="lblTime"></asp:Label>
</ContentTemplate>
</asp:UpdatePanel>
</div>
</form>
</body>
</html>
Default.aspx.cs
public partial class UpdatePanel_01_Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
/// Make btnShowTime postback asynchronously
this.ScriptManager1.RegisterAsyncPostBackControl(btnShowTime);
}
protected void btnShowTime_Click(object sender, EventArgs e)
{
lblTime.Text = DateTime.Now.ToLongTimeString();
}
}
WebMethods and PageMethods
Using WebMethods
By attaching the ScriptService and ScriptMethod attributes to your WebServices you can enable them to be accessed from within your pages via JavaScript.
Here’s a sample WebService with the ScriptService and ScriptMethod attributes attached:
[System.Web.Script.Services.ScriptService]
[WebService(Namespace = "<a href=/"http://tempuri.org/">http://tempuri.org/</a>/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
public class WSTime : System.Web.Services.WebService
{
[WebMethod]
[System.Web.Script.Services.ScriptMethod]
public string GetServerTime()
{
return DateTime.Now.ToLongTimeString();
}
}
Then to access the WebService from within JavaScript you need to first tell the ScriptManager about the WebService by giving it a ServiceReference. This can be done declaratively within the page, like so:
<asp:ScriptManager runat="server" ID="ScriptManager1">
<Services>
<asp:ServiceReference Path="~/WebMethods/WSTime.asmx" />
</Services>
</asp:ScriptManager>
Now to acess the WebServices methods you just use the namespace that ASP.NET Ajax creates in the page for the WebService and access its methods like normal JavaScript methods. The namespace that is created is the same name as the WebService, in this case it’s WSTime. The whole Ajaxiness of calling the server and getting the results back are handled for you, and all you have to worry about is processing the results. Remember, WebMethods can only be called Asynchronously.
Here’s an example of using the WSTime.GetServerTime WebService method from within JavaScript:
function btnGetServerTime_Click()
{
// call the WebMethod and pass it the method
// to call once it gets the response from
// the server.
WSTime.GetServerTime(SucceededCallback);
}
function SucceededCallback(result, eventArgs)
{
alert(result);
}
Using PageMethods
PageMethods are accessed in the same fashion as WebMethods, except they do not require the creation of a WebService. PageMethods are actually WebMethods that are added to the page itself. Once thing to note is there is No way to embed PageMethods within Custom User Controls or Server Controls, they must be added to the Page; hence the name PageMethods.
Here’s the C# code of an example page with a PageMethod defined:
public partial class PageMethods_Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
[WebMethod]
public static string GetServerTime()
{
return DateTime.Now.ToLongTimeString();
}
}
To enable the PageMethods you must first set the ScriptManagers EnablePageMethods property to True.
<asp:ScriptManager runat="server" ID="ScriptManager1" EnablePageMethods="true"></asp:ScriptManager>
Then you can call the PageMethods in the same way as WebMethods, except the namespace you use to access the methods is named PageMethods.
Here’s the example code of calling the above PageMethod from JavaScript:
function btnGetServerTime_Click()
{
PageMethods.GetServerTime(SucceededCallback);
}
function SucceededCallback(result, eventArgs)
{
alert(result);
}
Conclusion
I hope these couple of tips help in your endeavors with ASP.NET AJAX. When I get time (in between all the other million things) maybe I’ll post some more tips.
Also, if you have your own tips, please post them in the comments.
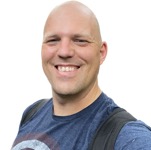
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.