Virtual Earth: Restrict Map to Specified Viewable Area
May 3, 2008 • Chris Pietschmann • MappingThis example demonstrates the ability to restrict the viewable area (and impose map boundaries) of a Virtual Earth map to be within a specified distance from the maps original center point (at time of initial load), and restrict to a maximum zoom level.
Why might you want to do this? There are times when you may want to restrict your users from possibly getting “lost” when viewing your data on a map. Also, you may want to restrict the user from panning around the globe in an attempt to reduce the number of Virtual Earth Map Transactions that you’ll end up paying for. Whatever the reasoning is; I’ve actually had this functionality requested a few times by clients, so I thought I’d write up a simple example.
This article was written with Virtual Earth v6.1, and will also work with Virtual Earth v6.0.
Download the Code:
VE_RestrictMapSpecifiedViewableArea.zip (3.01 kb)
Other Code Used In This Example
This example utilizes code that was included in the following two examples I’ve previously written:
Calculate Distance Between Geocodes in C# and JavaScript
Virtual Earth: Draw a Circle Radius Around a Lat/Long Point
Restrict Viewable Area
First create your map as usual; nothing special the creation of the VE map needed. Once you have your code in place to add the map to the page, you just need to handle the maps OnEndPan and OnStartPan events. You do this by attaching event handlers to these events, like the following:
//Attach our onendpan event handler
map.AttachEvent("onendpan", map_onendpan);
//Attach our onstartpan event handler
map.AttachEvent("onstartpan", map_onstartpan);
function map_onendpan(e) { }
function map_onstartpan(e) { }
Next, setup a couple global variables that will be used to define the restriction that will be imposed.
var mapRestrictionDistance = 100;
var mapRestrictionUnit = GeoCodeCalc.EarthRadiusInMiles;
The above two global variables define that you will restrict the map to only be panned within 100 miles of the maps original center point.
Next, you need to add code to the event handler method stubs shown above that will actually restrict the maps viewable area.
In the map_onstartpan event handler you will record the maps center point in a global variable before the panning begins. To do this add the following code:
// global variable to keep track of maps center point before panning began
var mapStartPanPoint = null;
function map_onstartpan(e)
{
//Get the current map center point before panning begins
mapStartPanPoint = map.GetCenter();
}
In the map_onendpan
event handler you will place the actual code that imposes our restriction. The following code will check if the map has been panned past the desired limitation of 100 miles from the original center point. If it has, it will reposition the map back to the last point the map was at before the invalid panning began. Here’s the code to implement this within the map_onendpan
event handler:
function map_onendpan(e)
{
//Get total distance panned from map center
var distance = GeoCodeCalc.CalcDistance(
mapOriginalCenterPoint.Latitude,
mapOriginalCenterPoint.Longitude,
map.GetCenter().Latitude,
map.GetCenter().Longitude,
mapRestrictionUnit
);
//Check distance panned from original center point
if (distance > mapRestrictionDistance)
{
//Move map back to the last point that was
//within the desired restriction radius
map.SetCenter(mapStartPanPoint);
}
}
Restrict Maximum Zoom Level
To add the Maximum Zoom Level restriction all you need to do is implement a handler for the maps OnEndZoom
event.
Use the following code to attach an event handler to the OnEndZoom
event:
//Attach our onendzoom event handler
map.AttachEvent("onendzoom", map_onendzoom);
function map_onendzoom(e) { }
Before you write the code that will place a restriction on the maximum zoom level, you need to declare a global variable that will define what the maximum zoom level will be.
var mapRestrictionZoomLevel = 7;
Now that you have the global variable in place, and the event is being handled by the map_onendzoom
method stub, you need to add the following code to the method that will put the zoom level restriction in place:
function map_onendzoom(e)
{
//Check if the map is zoomed out further than
//the set restriction
if (e.zoomLevel < mapRestrictionZoomLevel)
{
//Zoom the map back in to the restricted area
map.SetZoomLevel(mapRestrictionZoomLevel);
}
}
Conclusion
This is actually some rather simple code to write (at least when utilizing the GeoCodeCalc class) and it provides some good functionality to keep your users from possibly getting lost, along with potentially reducing the number of Virtual Earth Map Transactions you’ll end up paying for.
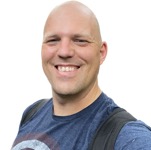
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.