Implementing Namespaces in Ruby using Nested Modules
Nov 2, 2008 • Chris Pietschmann • RubyRecently, I finally got around to starting to learn Ruby. I am finding it to be rather pleasant to program in. Now, one thing that I am used to using with an Object Oriented language (especially with my .NET background) are Namespaces. The only problem is that you can’t just declare a namespace in Ruby using a “namespace” keyword. However, it’s not difficult to implement Namespaces in Ruby, all you need to do is nest some modules.
To demonstrate this, I’ll show you how to create the familiar (for .NET developers) System.Windows.Forms namespace with the MessageBox.Show method:
module System
module Windows
module Forms
module MessageBox
def MessageBox.Show(title, message)
api = Win32API.new("user32","MessageBox",["L","P","P","L"],"I")
api.call(0, message, title, 0)
end
end
end
end
end
Now to use the namespace you can use one of the following methods:
System::Windows::Forms::MessageBox.Show("Some Title", "This is a test message.")
Or by including the “namespace”:
include System::Windows::Forms
MessageBox.Show("Some Title", "This is another test message.")
As you can see it’s rather simple to create “.NET”-like namespaces in Ruby.
Now, I know this technique is basically allowing me to write .NET code in Ruby, but that was pretty much the point of figuring this out. Now, back to learning how to write Ruby code in Ruby.
Oh, yeah now only if the syntax highlighter in BlogEngine.NET supported Ruby….
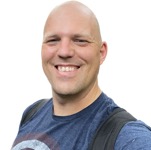
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.