Bing Maps Ajax 7: Simple Pushpin Animation via Extension to Microsoft.Maps
Nov 30, 2010 • Chris Pietschmann • MappingWhen updating the location a pushpin using the Bing Maps Ajax Control, the pushpin instantly moves to its new location. Sometimes this may work, but other times it may be nice to animate the movement. Updating the location a vehicle in an asset tracking application is one example where it would be nice to animate the movement.
I wrote a simple extension to the Bing Maps Ajax v7 control that adds the Pushpin.moveLocation
method to perform such an animation.
Usage
Let’s start with some sample usage of the Pushpin.moveLocation
method.
// Move pushpin to new location
// using the default animation speed
// of 1000 milliseconds (1 second)
pushpin.moveLocation(
new Microsoft.Maps.Location(47, -87)
);
// Move pushpin to new location
// using a speed of 2000 milliseconds
// or 2 seconds
pushpin.moveLocation(
new Microsoft.Maps.Location(47, -87),
2000
);
Method Reference
Here’s some documentation for the Pushpin.moveLocation
method:
Pushpin.moveLocation(location, speed)
This methods moves the pushpin to a new location using an animation of slowly moving the pushpin over the period of the specified interval.
- location: The Location to move the pushpin to.
- speed: The time period to perform the animation, in milliseconds. If not specified, the default is 1000 milliseconds, or 1 second.
Full Extension Source Code
Here’s the full source code for adding the Pushpin.moveLocation
extension to Bing Maps Ajax v7.
(function ($m) {
(function(proto) {
if (!proto.moveLocation){
proto.moveLocation = function(loc, speed) {
// loc = Location to move the pushpin to
// speed = time (in milliseconds) to perform animation
var that = this, startLoc = this.getLocation(),
endLoc = loc, startTime = new Date();
if (speed === undefined){
speed = 1000; // Default to 1 second
}
var interval = window.setInterval(function() {
var timeElapsed = (new Date()) - startTime;
if (timeElapsed >= speed){
// Full animation time (speed) has elapsed
// Just set final location and end animation
that.setLocation(endLoc);
window.clearInterval(interval);
}
// Set the Latitude/Longitude values to a percentage
// of the total distance to move based on the amount
// of time that has elapsed since animation started.
var timeElapsedPercent = (timeElapsed / speed);
var latitudeDistToMove = (
endLoc.latitude - startLoc.latitude
);
var longitudeDistToMove = (
endLoc.longitude - startLoc.longitude
);
that.setLocation(new $m.Location(
startLoc.latitude + (timeElapsedPercent * latitudeDistToMove),
startLoc.longitude + (timeElapsedPercent * longitudeDistToMove)
));
}, 10);
};
}
})($m.Pushpin.prototype);
})(Microsoft.Maps);
Conclusion
This is a really simple Pushpin animation extension that does its job very nicely. However, this is just the beginning of what a more feature full extension would provide.
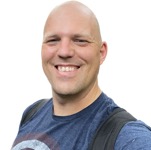
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.