C#: What's the Difference Between `int`, `var`, and `dynamic`?
Apr 9, 2025 • Chris Pietschmann • C#In C#, understanding the distinctions between int
, var
, and dynamic
is crucial for writing clear and efficient code. Each serves a unique purpose and behaves differently during compilation and runtime. Let’s explore these differences.
int
: The Explicit Integer Type
The int
keyword in C# is a straightforward, explicit declaration for 32-bit integer variables. When you declare a variable as int
, you’re specifying its type directly, and the compiler enforces this type strictly.
int number = 42;
In this case, number
is explicitly typed as an integer, and any attempt to assign a non-integer value to it will result in a compile-time error.
var
: Implicitly Typed Variables
The var
keyword allows for implicit typing. The compiler determines the type of the variable based on the assigned value at compile time. It’s important to note that var
is still statically typed; once the type is inferred, it cannot change. Essentially, var
enables you to rely on the compiler to help you write the code more efficiently; rather than explicitly.
var message = "Hello, World!"; // Compiler infers 'message' as string
var number = 42; // Compiler infers 'number' as int
Here, message
is inferred to be of type string
, and number
is inferred to be of type int
at compile time. Attempting to assign a value of a different type later will cause a compile-time error.
Key Points:
- The type must be determinable at the point of declaration.
- Once inferred, the type is fixed and cannot be changed.
- Improves code readability by reducing redundancy, especially with complex types.
dynamic
: Runtime Type Resolution
The dynamic
keyword in C# defers type checking until runtime. This means that variables declared as dynamic
can bypass compile-time type checking, offering greater flexibility but at the cost of type safety. The dynamic
keyword is the basis for being able to write dynamically typed code in C#.
dynamic value = 10;
value = "Now I'm a string"; // No compile-time error
In this example, value
is initially assigned an integer and later assigned a string. The compiler does not enforce type constraints on dynamic
variables, leading to potential runtime errors if the assigned values are incompatible with the expected operations.
Key Points:
- Type resolution occurs at runtime, not at compile time.
- Useful for interoperability with dynamic languages, COM objects, or scenarios where type information is not available at compile time.
- Lacks compile-time type checking, which can lead to runtime errors if not handled carefully.
Comparing int
, var
, and dynamic
Aspect | int |
var |
dynamic |
---|---|---|---|
Type Checking | Compile-time | Compile-time | Runtime |
Type Declaration | Explicit | Implicit (inferred at compile time) | Explicit |
Flexibility | Fixed type | Fixed type once inferred | Can change type during runtime |
IntelliSense Support | Full | Full | Limited (due to runtime type resolution) |
Error Detection | Compile-time | Compile-time | Runtime |
Best Practices
-
Use
int
(or other explicit types) when the type is known and clarity is paramount. Explicit typing enhances readability and maintainability. -
Use
var
to simplify code, especially when dealing with complex types or when the type is evident from the context. However, avoid usingvar
when the type is not apparent, as it can reduce code clarity. -
Use
dynamic
sparingly and only when necessary, such as when interacting with dynamic languages, COM objects, or frameworks that require runtime type flexibility. Be cautious, as it sacrifices compile-time type safety.
Understanding these distinctions ensures that you can choose the appropriate type declaration for your variables, leading to more robust and maintainable C# applications.
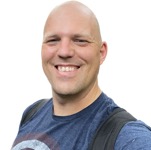
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.