C#/VB.NET: Extension Methods
Mar 13, 2007 • Chris Pietschmann • C# • Visual Basic • .NETOver a year ago I posted a question about doing a SQL-like IN operation in .NET. Here’s the examples I posted of how it could work:
Dim arrNames() AS String = New Array{"CHRIS", "TOM", "TYLER"}
If strName IN arrNames Then
'do something
End If
Now, this would be a powerful feature since you wouldn’t be required to write code that loops through the array or collection. Well, I saw some good news today posted by Scott Guthrie.
Scott posted about a new feature in .NET “Orcas” (I believe it’s going to be v3.5 but I’m note sure.), it’s called Extension Methods.
Extension Methods allow you (the developer) to add new functionality to existing .NET object type. And, you can do it without inheritance or recompiling the original type. This means you can add your own custom functionality to any third party object or framework object you want. This sounds really neat!
I saw this, and I thought to myself, Sweet you will now be able to do something similar to a SQL IN operation just as easily as it’s done in SQL. In fact, Scott even posted an example of how to do it. (however, he didn’t compare it to SQL IN like I am.)
The code for the IN method goes like this:
namespace SqlInMethodExtension
{
public static class SqlInMethodExtension
{
public static bool IN(this object o, IEnumerable c)
{
foreach (object i in c)
{
if (i.Equals(o)) return true;
}
return false;
}
}
}
Now using the method is as simple as this:
using SqlInMethodExtension;
public class Test
{
void Test(string myValue)
{
string [] values = {"Chris","John","Sam"};
if (myValue.IN(values))
{
// Do something here
}
}
}
Isn’t that cool!?
You’ve probably read about the new LINQ features that are also coming in .NET “Orcas”. According to ScottGu LINQ uses Extension Methods pretty heavily. So, if you ever wonder why this feature is being added, just think about the coolness of LINQ.
To read more about Extension Methods:
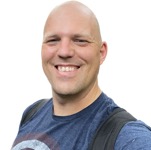
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.