C#: What is the difference between 'string' and System.String?
Apr 16, 2024 • Chris Pietschmann • C#In C#, understanding the nuances between string
and System.String
is helpful when writing efficient and maintainable code. This may seem like a non-issue, and in most cases it really isn’t that important to understand. However, there are times when understanding the differences and relationship between string
and System.String
can be important.
While they might seem interchangeable at first, they serve distinct purposes and have implications for coding practices. This article looks at the differences between them and explains the best practices associated with the usage of each.
What is string
?
In C#, ‘string’ is a keyword that represents a data type used to store a sequence of characters. It’s one of the most commonly used data types and is employed extensively for manipulating textual data. When you declare a variable using the ‘string’ keyword, you’re essentially creating an instance of the System.String class.
string str1 = "Hello World!";
Also, when using the var
keyword, the C# compiler will auto-detect the type appropriately. Here’s an example using var
that will compile to identical code to the above example:
var str1 = "Hello World!";
What is System.String
?
On the other hand, System.String is a class in the .NET Framework that represents a string of characters. It provides various methods and properties for string manipulation and comparison. In C#, when you use the string
keyword, you’re implicitly utilizing the System.String
class behind the scenes.
System.String str2 = "Hello World!";
If the code includes a using
reference to the System
namespace, then String
(with the capital S
) can be used instead of System.String
. Here’s an example of this:
String str2 = "Hello World!";
How are they used?
Both string
and System.String
can be used interchangeably to define variables that store text data. In fact, you’ll see in documentation and tutorials the use of string
as the way to declare a string variable.
Here’s a simple example illustrating the usage of both:
string str1 = "Hello"; // Using 'string' keyword
System.String str2 = "World"; // Using System.String class directly
Most commonly you’ll use and see the string
keyword used rather than explicitly using the System.String
class. In fact, the use of string
is actually a shorthand for System.String
. As a result, when the C# compiler encounters string
, it will automatically use the System.String
class.
Since, these can be used interchangeable, you can easily assign a string
or System.String
variable to one declared with the other seamlessly. Here’s an example of this:
string str1 = "Hello";
System.String str2;
str2 = str1;
// str2 will equal "Hello"
str2 = str1 + " World!";
// str2 will equal "Hello World!"
Tip: In C#, the
string
keyword is an alias forSystem.String
that the C# compiler automatically knows how to interpret.
Casting from one to the other can be done as well. However, while this may not be very practical in your code, it’s still possible to do. Here’s an example of this:
var str1 = "Hello";
System.String str2 = (string)str1;
str1 = (System.String)str2;
Since C# is case sensitive, remember that string
(all lowercase) and String
(with capital S
) are two different declarations. Here’s an example of this:
// include the `System` namespace
using System;
// lowercase is `string` shorthand
string = str1 = "Hello";
// capitalized `S` is shorthand for `System.String`
String str2 = "World";
Why do they both exist?
The existence of both string
and System.String
can be attributed to syntactic sugar and historical reasons. The string
keyword provides a convenient shorthand for declaring string variables without explicitly referencing the System.String
class. It enhances readability and reduces verbosity in code. On the other hand, System.String
represents the underlying implementation of strings in .NET, and it also exposes various methods and operations for string manipulation.
Best Practice Recommendation
While both string
and System.String
can be used interchangeably, it’s generally recommended to stick with the string
keyword for consistency and improved readability. Using string
aligns with common coding conventions and makes the code more understandable for other developers. Additionally, it allows the C# compiler to abstract away the implementation details of the System.String
class. It’s best to rely on syntactical sugar like this, so you can benefit from the code improvements that the C# compiler may offer.
Best Practice: It’s best practice to use the
string
keyword when declaring string variables ifvar
is not being used.
However, there might be rare scenarios where you need to explicitly use System.String
, such as when dealing with reflection or interoperating with legacy code. In these cases, it’s acceptable to use System.String
, but it’s best to be sure to only do so if absolutely necessary.
Conclusion
While string
and System.String
may seem like two sides of the same coin, understanding their differences and knowing when to use each can be helpful for writing clean, maintainable, and efficient C# code.
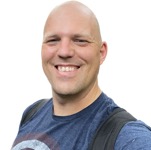
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.