Bing Maps Silverlight CTP: Adding Media (Images, Video, etc.) to the Map
Mar 20, 2009 • Chris Pietschmann • Mapping • SilverlightThis was written for the Bing Maps Silverlight CTP Release.
In the previous tutorial (“Basics of Adding Polygons and Polylines using XAML and Code”) I mentioned that there is no Pushpin Shape Type in the new Virtual Earth Silverlight Map Control SDK CTP. Instead, the Silverlight control has the flexibility of plotting/displaying anything (as long as it inherits from UIElement) you want at a specific Latitude/Longitude Coordinate. This opens up a ton of UI options that can be built to allow the user to interact with the data being displayed.
One thing to keep in mind when adding Images, Video and any other UIElements to the Map is that you’ll need to add them to a MapLayer, then add the MapLayer to the Map. This is required so that you can specify the Location to Plot the UIElement at. This is something that you’ll probably want to do anyway so you can easily control the layering of the elements that are plotted, or even easily show/hide groups of elements (by having a seperate MapLayer contain the elements for each specific group.)
Let’s start plotting some cool stuff on a Map. ## Adding Images to the Map (aka “Pushpins”)
When adding Images to the Map, the process is very similar to adding Polygons or Polylines, except you need to add Images to a MapLayer first, then add the MapLayer to the Map.
The closest thing with the Silverlight Map Control to the “Pushpins” of the old JavaScript control is the ability to directly plot Images on the Map.
// Create MapLayer
MapLayer myLayer = new MapLayer();
// Create Image
Image image = new Image();
// Set the Image Source
image.Source = new BitmapImage(new Uri(“pushpinImage.png”, UriKind.Relative));
// Set Image Display Properties
image.Opacity = 0.8;
image.Stretch = Stretch.None;
// Create a Location object that defines where the “Shape” will be plotted
Location location = new Location() { Latitude = -10, Longitude = 10 };
// Center the image around the location specified
PositionMethod position = PositionMethod.Center;
// Add Image to the Layer
myLayer.AddChild(image, location, position);
// Add Layer to the Map
Map1.AddChild(myLayer); ## Adding Video to the Map
Plotting Video content on the Map is a good example of what some of the possibilities are opening up with this new Virtual Earth Silverlight Map Control. To do this all you need to do is create a MediaElement with its Source set to the Uri of a Video and add it to the Map in the same way as shown above with adding Images to the Map.
// Create MapLayer
MapLayer myLayer = new MapLayer();
// Create MediaElement
MediaElement video = new MediaElement();
// Set the MediaElement Source to the Video
video.Source = new Uri(“http://mschnlnine.vo.llnwd.net/d1/ch9/8/9/1/1/6/4/ScottGuthrieSL3_ch9.wmv”,
UriKind.RelativeOrAbsolute);
// Set MediaElement Display Properties
video.Opacity = 0.8;
video.Width = 200;
video.Height = 150;
// Create a Location object that defines where the “Shape” will be plotted
Location location = new Location() { Latitude = -10, Longitude = 10 };
// Center the image around the location specified
PositionMethod position = PositionMethod.Center;
// Add Image to the Layer
myLayer.AddChild(video, location, position);
// Add Layer to the Map
Map1.AddChild(myLayer); ## Conclusion
You can add any visual control/object that derives from UIElement to the Map using the same method described above with Images and Video. This really opens up the possibilities to plot/add any kind of Rich Content to the Map. The possibilities are almost endless. ### Next Tutorial/Article: Using Tile Layers to Overlay Custom Map Imagery
### Previous Tutorial/Article: Basics of Adding Polygons and Polylines using XAML and Code
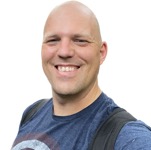
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.