CTP: Bing Maps Silverlight CTP: Basics of Adding Polygons and Polylines using XAML and Code
Mar 20, 2009 • Chris Pietschmann • Mapping • SilverlightThis was written for the Bing Maps Silverlight CTP Release.
Now that we’re familiar with the basics of the Virtual Earth Silverlight Map Control SDK CTP (from my Getting Started with Virtual Earth Silverlight CTP tutorial), we’re ready to move on to adding Shapes (Polygons and Polylines). After all what use is a Virtual Earth Map Control without plotting some data on it. If you’re not familiar with how to get a Map displayed within a Silverlight Application, I really encourage you to go take a look at my “Getting Started with Virtual Earth Silverlight Map Control SDK CTP” tutorial.
Did you notice that I left out Pushpins? Well, this is actually because the Map control doesn’t have a Pushpin Shape Type; the only Shapes Types are Polygon and Polyline. The reason for this is because instead of limiting you to just Pushpins (a small icon of some sort) like the JavaScript Map Control; the Silverlight Control allows you to plot/display anything (as long as it inherits from UIElement) you want at a specific Latitude/Longitude Coordinate. Since, “Pushpins” are a newly changed concept with the Silverlight Map Control; I’ll be covering that is a separate tutorial that covers “Adding Media (Images, Video, etc.) to the Map”.
Now let’s get to plotting some Polygons and Polylines!
Adding Polygons to the Map
To add a Polygon to the Map, you will need to create an instance of the MapPolygon object and add a collection of Location objects to its “Locations” property. You will also need to define the look of your Polygon by setting its “Fill”, “Stroke”, “StrokeThickness” and “Opacity” properties.
The MapPolygon properties and what they do:
- **Fill** – A Brush object that defines how the inside of the Polygon is filled in.
- **Stroke** – A Brush object that defines how the border that outlines the Polygon will be drawn.
- **StrokeThickness** – A Double value that defines the thickness of the border that outlines the Polygon.
- **Opacity** – A Double value that defines the Opacity (or Transparency) of the Polygon.
When adding Polygons to the Map you add your MapPolygon object instance to the Map.Children collection.
Add a Polygon Programmatically using Code
Here’s an example of adding a Polygon to a Map programmatically:
MapPolygon polygon = new MapPolygon();
polygon.Fill = new SolidColorBrush(Colors.Red);
polygon.Stroke = new SolidColorBrush(Colors.Yellow);
polygon.StrokeThickness = 5;
polygon.Opacity = 0.7;
polygon.Locations = new LocationCollection()
{
new Location(20, -20),
new Location(20, 20),
new Location(-20, 20),
new Location(-20, -20)
};
Map1.AddChild(polygon);
// Map1.Children.Add(polygon); // <—This also works just the same
Add a Polygon Declaratively using XAML
One other neat thing that you can do is add Polygons to a Map declaratively using XAML. Here’s an example:
Adding Polylines to the Map
To add a Polyline to the Map, you will need to create an instance of the MapPolyline object and add a collection of Location objects to its “Locations” property. You will also need to define the look of your Polyline by setting its “Stroke”, “StrokeThickness” and “Opacity” properties.
The MapPolyline properties and what they do:
- **Stroke** – A Brush object that defines how the Line that represents the Polyline will be drawn on the Map.
- **StrokeThickness** – A Double value that defines the thickness of the Line.
- **Opacity** – A Double value that defines the Opacity (or Transparency) of the Polyline.
Additionally, the MapPolyline object has one more property that I didn’t mention above. This is a little bit of a special property, that you may wonder why a Polyline object has it, but it opens up some possibilities that weren’t available before using the JavaScript Map Control.
- **Fill** - A Brush object that defines how the inside of the Polyline is filled in. This will connect the first and last Location point of the Polyline and Fill in between using the designated Brush. This doesn’t draw a Line (or Stroke) from the first Location to the last, it just fills in between the Shape.
When adding Polylines to the Map you add your MapPolyline object instance to the Map.Children collection; just the same as is done with Polygons.
Add a Polyline Programmatically using Code
Here’s an example of adding a Polyline to a Map programmatically:
MapPolyline polyline = new MapPolyline();
polyline.Stroke = new SolidColorBrush(Colors.Yellow);
polyline.StrokeThickness = 5;
polyline.Opacity = 0.7;
polyline.Locations = new LocationCollection()
{
new Location(10, -10),
new Location(10, 10),
new Location(-10, -10),
new Location(-10, 10)
};
Map1.AddChild(polyline);
// Map1.Children.Add(polyline); // <—This also works just the same
Add a Polyline Declaratively using XAML
One other neat thing that you can do is add Polylines to a Map declaratively using XAML. Here’s an example:
Adding Shape Layers
In the old JavaScript Map Control, you could add your Shapes to different Shape Layers, then you could easily hide/show the Shapes in each Layer by hiding/showing the entire Shape Layer. This is also possible using the Virtual Earth Silverlight Map Control via the MapLayer object.
To use the MapLayer object you simply create a new MapLayer, add your Shapes (Polygons or Polylines) to it, then add the MapLayer to the Map.
Here’s an example of doing this:
// Create MapLayer
MapLayer myShapeLayer = new MapLayer();
// Create Polygon
MapPolygon polygon = new MapPolygon();
polygon.Fill = new SolidColorBrush(Colors.Red);
polygon.Stroke = new SolidColorBrush(Colors.Yellow);
polygon.StrokeThickness = 5;
polygon.Opacity = 0.7;
polygon.Locations = new LocationCollection()
{
new Location(20, -20),
new Location(20, 20),
new Location(-20, 20),
new Location(-20, -20)
};
// Add Polygon to the Layer
myShapeLayer.AddChild(polygon);
// Add the Layer to the Map
Map1.AddChild(myShapeLayer);
Easily Add a “Hover Over” Effect Via Tooltips
If you want to quickly and easily add some content that gets displayed when the user hovers the mouse over the Polygon or Polyline; you can implement it very easily by taking advantage of Silverlights Tooltip feature.
Here are some code examples of doing this that add to the above Polygon examples (the exact same can be done with Polylines).
Add Tooltip Declaratively using XAML
Add Tooltip Programmatically using Code
// Declare Polygon
MapPolygon polygon = new MapPolygon();
polygon.Fill = new SolidColorBrush(Colors.Red);
polygon.Stroke = new SolidColorBrush(Colors.Yellow);
polygon.StrokeThickness = 5;
polygon.Opacity = 0.7;
polygon.Locations = new LocationCollection()
{
new Location(20, -20),
new Location(20, 20),
new Location(-20, 20),
new Location(-20, -20)
};
// Add Tooltip
var tooltipObject = new StackPanel();
var title = new TextBlock();
title.FontWeight = FontWeights.Bold;
title.Text = “A Huge Square Title”;
tooltipObject.Children.Add(title);
var description = new TextBlock();
description.Text = “This is an arbitrary description of the "Huge Square" to be displayed within the Tooltip.”;
tooltipObject.Children.Add(description);
ToolTipService.SetToolTip(polygon, tooltipObject);
// Add Polygon to Map
Map1.AddChild(polygon);
// Map1.Children.Add(polygon); // <—This also works just the same
Conclusion
As you can see, it’s fairly simple to add Polygons and Polylines to the Map. In the next tutorial I’ll cover how to add Image (also “Pushpins”) and Video to the Map.
Next Tutorial/Article: Adding Media (Images, Video, etc.) to the Map
Previous Tutorial/Article: Getting Started with Virtual Earth Silverlight Map Control SDK CTP
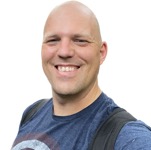
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.