Scrape Geocodes from Google Maps w/ C#
Jun 13, 2006 • Chris Pietschmann • Mapping • C#I need to geocode some addresses once in a while and I notice if you view the source of the Google Maps page, the geocodes are right there. So instead of looking at the source of the page manually, I just created a little app that scrapes them from the page for me and places them into textboxes.
Below is a small code snippet I wrote that does just that. It’s not perfect but it is simple and it just plain works.
string lat = "";
string lng = "";
string address = "1 Microsoft Way, Redmond WA";
try
{
System.Net.WebClient client = new System.Net.WebClient();
string page = client.DownloadString("http://maps.google.com/maps?q=" + address);
int begin = page.IndexOf("markers: [");
string str = page.Substring(begin);
int end = str.IndexOf(",image:");
str = str.Substring(0, end);
//Parse out Latitude
lat = str.Substring(str.IndexOf(",lat: ") + 6);
lat = lat.Substring(0, lat.IndexOf(",lng: "));
//Parse out Longitude
lng = str.Substring(str.IndexOf(",lng: ") + 6);
}
catch (Exception ex)
{
MessageBox.Show("An Error Occured Loading Geocode!\nCheck that a valid address has been entered.", "An Error Occured Loading Geocode!");
}
MessageBox.Show("Latitude:\n" + lat + "\n\nLongitude:\n" + lng);
I know I should have used Regular Expressions, but I don’t know them very well and this was just quicker/easier to do. It took all of like 5 minutes.
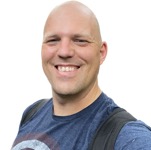
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.