C#: Enhance Enums using Extension Methods
Jul 15, 2008 • Chris Pietschmann • C# • .NETExtension Methods are one of the coolest features that have been added in .NET 3.5. I’ve heard arguments that there is no reason to use them, and the only reason Microsoft added them is to enable the ability to buid LINQ. Well, I do not entirely agree with that statement; in fact, I have found a cool way to use Extension Methods to enhance the System.Enum object since it cannot be inherited. Even though Enum can not be inherited, it can be extended using Extension Methods.
Here’s some example code for a simple Enum that has a DescriptionAttribute applied to each of it’s values:
public enum LocalizationMarket
{
[Description("en-US")]
English = 1,
[Description("en-ES")]
Spanish = 2
}
And here’s the code to an Extension Method that extends the LocalizationMarket Enum with the ToDescriptionString() method that returns the DescriptionAttributes value:
public static class LocalizationMarketExtensions
{
public static string ToDescriptionString(this LocalizationMarket val)
{
DescriptionAttribute[] attributes = (DescriptionAttribute[])val.GetType().GetField(val.ToString()).GetCustomAttributes(typeof(DescriptionAttribute), false);
return attributes.Length > 0 ? attributes[0].Description : string.Empty;
}
}
The usage of this new method is really simple:
LocalizationMarket myLocal = LocalizationMarket.English;
// this will show "en-US" in the MessageBox that's shown
MessageBox.Show( myLocal.ToDescriptionString() );
Now one thing you must remember with using Extension Methods is you may not want to extend the System.Enum Type, but instead just extend the Enums you create only.
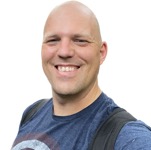
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.