HTML5 Day 3: Detecting HTML5 Support via JavaScript
Nov 16, 2010 • Chris Pietschmann • HTML • JavascriptOn Day 1, I covered exactly what HTML5 is and what’s necessary to convince older web browsers to render/style the new tags properly. Once you told the browser how to style the element, it still wont show special UI for the user. The good news is that you can “patch” the browser using JavaScript to enable such functionality. In this post we’ll discuss how to detect if a certain feature is supported by the browser or not.
Why is the UserAgent unreliable for detection?
Mozilla/4.0 (compatible; MSIE 8.0; Windows NT 6.1; WOW64;
Trident/4.0; SLCC2; .NET CLR 2.0.50727; .NET CLR 3.5.30729;
.NET CLR 3.0.30729; Media Center PC 6.0; .NET4.0C; .NET4.0E;
InfoPath.3; MS-RTC LM 8; Zune 4.7) </pre>
You could always check the UserAgent of the request on the web server and return the necessary javascript accordingly, however, that would require a tremendous amount of work. You would need to figure out what HTML5 features are supported by each version of each web browser that a user might use. That would be a tremendous nightmare.
So the only reliable method is to use a little JavaScript code to do the detection on the client-side. This way you can check if each feature is supported before executing your “patch” code to add support.
Individual Attribute Detection
Lets take the following as an example:
<input type="text" placeholder="enter value" />
One of the new features in HTML5 is the “placeholder” attribute on the <input>
tag. This attribute allows you to show some specified text (as a watermark) in the input box until the user enters a value.
Let’s imagine we want to add our own “placeholder” functionality in older web browsers that do not natively support this feature using a JavaScript “patch”. Before we “patch” the browser, we must first detect whether this feature is already supported. To do this, we can use the following JavaScript code:
function IsAttributeSupported(tagName, attrName) {
var val = false;
// Create element
var input = document.createElement(tagName);
// Check if attribute (attrName)
// attribute exists
if (attrName in input) {
val = true;
}
// Delete "input" variable to
// clear up its resources
delete input;
// Return detected value
return val;
}
if (!IsAttributeSupported("input", "placeholder")) {
// Do something special here
alert("placeholder attribute is not supported");
}
You can use the above code with any attributes you want to detect on any element, just change the “input” and “placeholder” within the method call accordingly.
Input Type Detection
There are a number of new input types added in HTML5. The good news is that older browsers will render any unknown types as type=”text”. A couple of the new types are:
<input type="date" />
<input type="email" />
Let’s imagine we want to add you own UI to allow the user to select the date from a date picker control and the email from a list of contacts in older browsers that do not support these features natively. First we must detect if the browser supports these input types. To do this, we can use the following JavaScript code:
funciton IsInputTypeSupported(typeName) {
// Create element
var input = document.createElement("input");
// attempt to set the specified type
input.setAttribute("type", typeName);
// If the "type" property equals "text"
// then that input type is not supported
// by the browser
var val = (input.type !== "text");
// Delete "input" variable to
// clear up its resources
delete input;
// Return the detected value
return val;
}
if (!IsInputTypeSupported("date")) {
// Do something special
alert("date input is not supported");
}
if (!IsInputTypeSupported("email")) {
// Do something special
alert("email input is not supported");
}
Now you can execute your own “patch” in older browsers to add custom UI to these input fields.
While there are some exceptions in certain browsers, the above code will work for basic feature detection. One such exception is that the Chrome web browser supports the “date” input, but it doesn’t show any special UI for the user. This is an example where you may want to still show you one UI, but the above detection script will report that “date” inputs are supported in Chrome.
Modernizr Detection Script
You could detect HTML5 features yourself using the previous JavaScript code, but that is probably like using your own JavaScript code to perform animations instead of using jQuery. The Modernizr script does for feature detection as jQuery has done for the HTML DOM itself.
Here’s an example of using Modernizr to detect if the “date” input type is supported:
if (!Modernizr.inputtypes.date) {
// Do something special
alert("date input is not supported");
}
You can view the full Modernizr documentation for its full usage.
Additional Resources
- Dive into HTML5: Detecting HTML5 Features
- HTML5 Doctor: How to use HTML5 in your client work right now
Conclusion
Just like most things with JavaScript, it’s easy to perform basic feature detection. Although, you can get a lot of benefit of using a full framework to assist you in the process. Just as I use jQuery over custom HTML DOM code in most cases, I am liking the HTML5 detection support that Modernizr adds.
In tomorrows post I’ll move on to adding some “patch” code to add some support for new features in old browsers now that feature detection is out of the way.
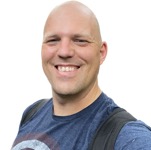
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.