HTML5 Day 4: Add Drop Down Menu to ASP.NET MVC HTML5 Template using CSS and jQuery
Nov 17, 2010 • Chris Pietschmann • HTML • ASP.NET • CSS • jQueryToday I was playing around with using jQuery to create a drop down menu. I thought I’d share an example of adding a drop down menu to the new ASP.NET MVC HTML5 Template that I created on Day 2.
Before we begin, you’ll need to create a new site using the code from Day 2. You can either copy/paste the code from the article, or download the full source code. You’ll find the download link at the bottom of the post for Day 2.
Here’s a screenshot of the finished dropdown menu:
Here’s another screenshot of the menu in Chrome:
Add Sub-Menu Items
First, modify the <nav>
item in the Site.Master file to include sub-menu items as <ul>
child elements added to the nav ul
element.
Below is an example:
<nav>
<ul>
<li><%: Html.ActionLink("Home", "Index", "Home")%></li>
<li>
<a href="#">Customers</a>
<ul>
<li><a href="#">Create New</a></li>
<li><a href="#">Latest</a></li>
<li><a href="#">View All</a></li>
</ul>
</li>
<li>
<a href="#">Orders</a>
<ul>
<li><a href="#">Create New</a></li>
<li><a href="#">Latest: Last 30 Days</a></li>
<li><a href="#">Search: Date Range</a></li>
<li><a href="#">Search: Customer</a></li>
<li><a href="#">View All</a></li>
</ul>
</li>
<li><%: Html.ActionLink("About", "About", "Home")%></li>
</ul>
</nav>
Of course don’t forget to replace the <a href
tags with Html.ActionLink
calls to wire up the Views in your application. For this article, we’ll just leave the code like this.
Add CSS Styles to the Sub-Menus
We do not need to modify the styles in the Site.css
file for this. We just need to add some CSS to style the sub-menus appropriately.
Here’s the minimum styles necessary for the sub-menu to display correctly:
body nav ul ul {
display: none;
position:absolute;
}
Even though the above style will make the sub-menus display correctly (hide on page load, and absolutely positioned), we still need to style them to match the overall style of the ASP.NET MVC site template.
Here’s the full CSS necessary to style the sub-menus in the new ASP.NET MVC HTML5 Template:
body nav ul ul {
display: none;
position:absolute;
width: 100%;
background: #5C87B2;
border-top: 5px solid #5C87B2;;
filter:alpha(opacity=80);
-moz-opacity:0.8;
-khtml-opacity: 0.8;
opacity: 0.8;
}
body nav ul ul li a{
line-height: 3.1em;
}
This CSS colors the background of the sub-menu area the same color blue as the page, but it also makes it semi-transparent. So any content behind the sub-menu will show through just a little bit.
Let’s wire things up with jQuery
Now we just need a little jQuery code to wire up our sub-menus to display when the use hovers the mouse over their parents. The jQuery code I’m using for this was inspired by Dan Wellman’s <a href="http://net.tutsplus.com/tutorials/html-css-techniques/how-to-create-a-drop-down-nav-menu-with-html5-css3-and-jquery/">How to Create a Drop-down Nav Menu with HTML5, CSS3 and jQuery</a>
post.
$(function () {
$("body nav li").each(function () {
if ($(this).find("ul").length > 0) {
//show subnav on hover
$(this).mouseenter(function () {
$(this).find("ul").stop(true, true).slideDown();
});
//hide submenus on exit
$(this).mouseleave(function () {
$(this).find("ul").stop(true, true).slideUp();
});
$(this).find("ul").mousemove(function () {
$(this).stop(true, true).show();
});
}
});
});
As you can see, with jQuery the javascript code necessary to wire this up is rather short. Oh, and did I mention that the sub-menus slide down into place?
Conclusion
I know this post isn’t so much HTML5 as it is jQuery and CSS. But, after all, isn’t HTML5 really about the same thing as jQuery? RIA.
I know, yesterday, I said that today I would move on to adding some patch
code to add some HTML5 support to older browsers. I decided to take a detour back to the ASP.NET MVC HTML5 template. We shall see, again, where tomorrow takes us.
Source Code: MvcHTML5DropDownMenu.zip
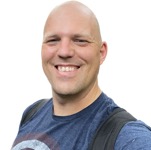
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.