jHash v2.0 Released: Now with Routing Support!
May 1, 2012 • Chris Pietschmann • Open SourcejHash is a small, lightweight (4kb minified / 2kb compressed) javascript library that makes it extremely easy to work with location.hash
. Version 1.0 had the ability to set / retrieve hash root values as well as “hash querystring” values. The newly released version 2.0 includes a new lightweight routing engine that facilitates an easier developer experience when building Single Page Applications.
The jHash documentation contains full descriptions of the libraries methods and their usage.
Get jHash Here: https://github.com/crpietschmann/jHash
Easily work with “location.hash”
Here are some simple examples of setting and retrieving hash “root” and “querystring” values:
// URL:
// http://localhost/page.htm#SomeValue?name=Chris&location=Wisconsin
// get "root" hash value
var root = jHash.root(); // returns "SomeValue"
// get "name" hash querystring value
var name = jHash.val('name'); // returns "Chris"
// get "location" hash querystring value
var loc = jHash.val('location'); // return "Wisconsin"
// set new individual query string value
jHash.val('name', 'Steve');
// set all new query string hash values
jHash.val({
name: 'Steve',
location: 'Montana'
});
Easy “location.hash” Routing with jHash
Hash routes are a technique that can be used to build Single Page Applications more easily. The Routing implementation contained within jHash works so you can declare Route Patterns in a similar fashion to ASP.NET Routes.
The “Using jHash Routes article in the jHash documentation” contains full descriptions and examples on how to use jHash Routes.
Here’s a short code sample that shows how to add a simple hash route handler and a sample Hash value that will match the route specified:
// Hash that would match this Route Pattern
// #Wisconsin/Milwaukee
jHash.route('{state}/{city}',
function () {
var stateName = this.state;
// stateName will equal 'Wisconsin'
var cityName = this.city;
// cityName will equal 'Milwaukee'
}
);
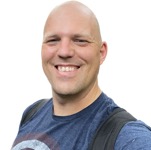
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.