Using the Popover API with HTML and Javascript in the browser
May 10, 2024 • Chris Pietschmann • HTML • JavascriptPopovers have become a ubiquitous UI element on the web, offering a clean and intuitive way to display additional information or actions to users. The Popover API provides native HTML features, with Javascript integration, to finally enabled developers an easier experience for implementing popover UI’s on the web. Let’s take a look at how to use the Popover API in the browser!
Understanding the Popover API
The Popover API provides developers with a standardized approach to create and manage popovers dynamically on web pages. Whether you need simple tooltips, complex menus, or interactive dialogs, the Popover API offers the flexibility to meet your requirements.
Creating Declarative Popovers
One of the simplest ways to create a popover is by leveraging HTML attributes. Adding the popover attribute to an element hides it initially, and you can control its visibility using designated control buttons. Here’s a basic example:
<button popovertarget="mypopover">Toggle the popover</button>
<div id="mypopover" popover>Popover content</div>
This code snippet creates a popover associated with a <div>
element and a toggle button to control its visibility.
Auto State and “Light Dismiss”
Popovers can have an “auto” state, enabling them to be dismissed by clicking outside or using browser-specific mechanisms like the Esc key. This behavior simplifies user interaction and ensures a seamless experience. Additionally, only one auto popover is shown at a time, preventing clutter on the screen.
Using Manual Popover State
Alternatively, you can opt for manual state, where popovers remain visible until explicitly closed. This mode allows for multiple independent popovers to be shown simultaneously, ideal for scenarios requiring more control over popover interactions.
<div id="mypopover" popover="manual">Popover content</div>
Using this, the popover cannot be “light dismissed”, it needs to be explicitly hidden. This also enables the use of multiple independent popovers at the same time.
Here’s an expanded example of this:
<button popovertarget="mypopover">Toggle the popover</button>
<div id="mypopover" popover="manual">
<h1>Popover content</h1>
<button popovertarget="mypopover" popovertargetaction="hide">
Hide popover
</button>
</div>
Also, here’s a live example you can try out in this article:
Popover content
Controlling Popovers via Javascript
The Popover API also provides a robust JavaScript interface for programmatic control. You can dynamically create popovers, define their behavior, and manage their visibility using JavaScript methods like showPopover()
and hidePopover()
. This enables advanced interactions and dynamic content generation based on user actions or application logic.
// Show popover
document.getElementById('mypopover').showPopover();
// Hide popover
document.getElementById('mypopover').hidePopover();
Additional Techniques for using Popover API
Dismissing Popovers Automatically via a Timer
A common use case involves dismissing popovers after a certain duration, similar to toast notifications. By combining manual popovers with JavaScript’s setTimeout() function, you can create self-dismissing popovers, enhancing user feedback and interaction.
var popover = document.getElementById('mypopover');
popover.showPopover();
setTimeout(() => {
popover.hidePopover();
}, 4000);
Styling and Animating Popovers
The Popover API offers extensive CSS customization options, allowing developers to style popovers to match their application’s design language. From positioning and background effects to animations and transitions, you can tailor popovers to seamlessly integrate into your UI.
Set default CSS styling of Popovers:
[popover] {
position: fixed;
inset: 0;
width: fit-content;
height: fit-content;
margin: auto;
border: solid;
padding: 0.25em;
overflow: auto;
color: CanvasText;
background-color: Canvas;
}
Override Popover CSS styling to display Popover in a different location in the viewport:
:popover-open {
width: 200px;
height: 100px;
position: absolute;
inset: unset;
bottom: 5px;
right: 5px;
margin: 0;
}
Style the ::backdrop
, a pseudo element shown full-screen directly behind the popover that’s being displayed in the top later of the page. This allows for background effects to be added, like the typical greying out or blurring of the background:
::backdrop {
backdrop-filter: blur(3px);
}
Exploring Nested Popovers
Nested popovers introduce a level of complexity by allowing multiple popovers to coexist within each other. This feature is particularly useful for creating nested menus or hierarchical interfaces. By understanding the different nesting techniques and event handling, you can implement sophisticated popover structures.
Conclusion
The Popover API is a great feature addition to HTML and Javascript that enables developers to create versatile and user-friendly interfaces enriched with interactive elements. Whether you’re building a simple alert prompt or a more complex scenario, the Popover API provides an easy to use API for a feature that was traditionally much more complicated to implement in the past.
Happy coding!
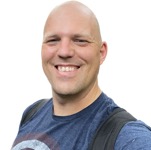
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.