CSS Image Styling Techniques
Jul 9, 2024 • Chris Pietschmann • CSSStyling images with CSS can significantly enhance your website’s visual appeal. In this guide, we’ll explore various techniques to transform your images using CSS, from creating circular shapes to applying filters. Let’s dive in and discover how to make your images stand out.
Rounded Corners
Rounded corners are a really common effect that is added to images on websites. You can achieve this by setting the border-radius
to a value indicating how big the rounded corner should be.
Slightly Rounded Corners
img {
border-radius: 0.5em;
/*
could also set using pixels instead:
border-radius: 5px;
*/
}
Large Rounded Corners
img {
border-radius: 2em;
/*
could also set using pixels instead:
border-radius: 30px;
*/
}
Circular Images
Creating circular images is a straightforward process. You can achieve this by setting the border-radius
property to 50%. Ensure the width and height of your image are equal to maintain a perfect circle.
img {
border-radius: 50%;
width: 200px;
height: 200px;
}
Alternatively, the clip-path
property can be used for more complex shapes.
img {
clip-path: circle(50%);
width: 200px;
height: 200px;
}
Centering Images
Centering images within their container can be done using the display
and margin
properties. Setting the image to block
display and applying margin: 0 auto
will center it horizontally.
img {
display: block;
margin: 0 auto;
width: 500px;
}
Responsive Images
To ensure your images adapt to different screen sizes, use the max-width
property. This makes the image resize itself according to the screen size while maintaining its aspect ratio.
img {
max-width: 100%;
height: auto;
}
For more control, media queries can adjust the image size for specific screen dimensions.
@media only screen and (max-width: 480px) {
img {
width: 100%;
}
}
Transparent Images
Adding transparency to images can create subtle effects. The opacity
property controls the transparency level, where 1 is fully opaque and 0 is fully transparent.
img {
opacity: 0.5;
}
Image Filters
CSS filters can dramatically change the appearance of images. Properties like brightness
, contrast
, and saturate
allow you to tweak visual aspects easily.
Brightness
img {
filter: brightness(150%);
}
Contrast
img {
filter: contrast(150%);
}
Saturate
img {
filter: saturate(150%);
}
Greyscale
img {
filter: grayscale(100%);
}
Sepia
img {
filter: sepia(100%);
}
Blur
img {
filter: blur(5px);
}
Invert
img {
filter: invert(100%);
}
Hue-Rotate
img {
filter: hue-rotate(90deg);
}
Drop Shadow
The drop-shadow
filter adds a shadow to the image, similar to the box-shadow
property but specifically for images and other elements.
img {
filter: drop-shadow(10px 10px 5px gray);
}
These transforms can be combined to create unique visual effects. For example, you can create a grayscale, blurred image with a shadow:
img {
filter: grayscale(100%) blur(5px) drop-shadow(10px 10px 5px gray);
}
Using these additional CSS transforms allows for a wide range of image styling possibilities, enabling you to enhance your web design creatively and effectively.
Text Over Images
Placing text over images can enhance their utility. Using a combination of CSS positioning and z-index
, you can overlay text on images.
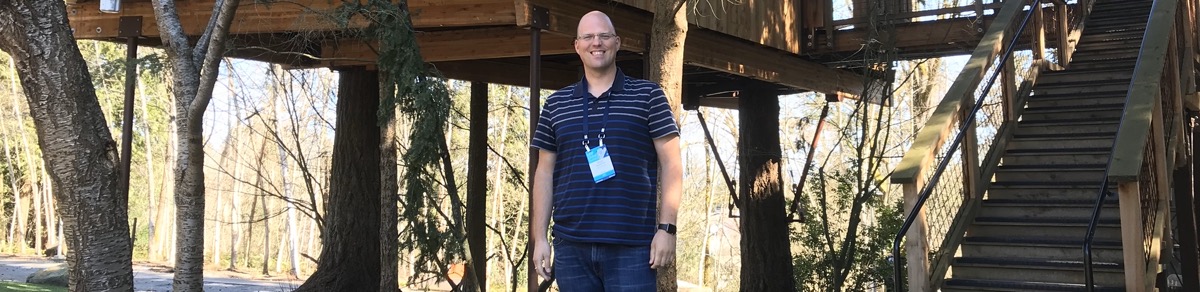
HTML
<div class="image-container">
<img src="image.jpg" alt="Descriptive text">
<div class="image-text">Your Text Here</div>
</div>
CSS
.image-container {
position: relative;
}
.image-text {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
z-index: 1;
color: white;
font-size: 20px;
font-weight: bold;
}
Flipping Images
Flipping images horizontally or vertically can add interesting visual effects. The transform
property with scaleX()
or scaleY()
functions achieves this.
Horizontal flip
img {
transform: scaleX(-1);
}
Vertical flip
img {
transform: scaleY(-1);
}
Conclusion
Mastering CSS image styling opens up numerous possibilities for web design. Whether you’re centering, resizing, or adding filters to your images, these techniques will help create visually appealing and responsive web pages.
These methods cover various aspects of CSS image styling, providing you with tools to enhance your website’s visuals effectively.
Happy styling!
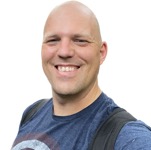
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.