Resetting Identity Seed in SQL Server After Deleting Records
Feb 3, 2025 • Chris Pietschmann • DatabaseIn SQL Server, identity columns generate auto-incremented values, ensuring uniqueness in a table. When records are deleted, the identity seed does not reset automatically. This can create gaps in the sequence, leading to unexpected behaviors in applications that depend on sequential numbering.
For example, consider the following table:
CREATE TABLE Employees (
ID INT IDENTITY(1,1) PRIMARY KEY,
Name VARCHAR(100)
);
If we insert three records and then delete them:
INSERT INTO Employees (Name) VALUES ('Alice'), ('Bob'), ('Charlie');
DELETE FROM Employees;
Adding a new record will result in an ID of 4
, not 1
, because the identity counter has not reset.
Solution: Using DBCC CHECKIDENT
The SQL Server command DBCC CHECKIDENT
allows you to reset the identity seed manually.
DBCC CHECKIDENT ('Employees', RESEED, 0);
This command resets the identity value to 0
, meaning the next inserted record will start at 1
.
'Employees'
: Specifies the table where the identity seed should be reset.RESEED
: Instructs SQL Server to reset the identity value.0
: Resets the counter so that the next inserted row gets1
.
Alternative: Using TRUNCATE TABLE
If you want to completely remove all records and reset the identity seed in one step, you can use TRUNCATE TABLE
:
TRUNCATE TABLE Employees;
Key Differences Between DELETE
and TRUNCATE
DELETE FROM Employees;
removes records but keeps the identity seed intact.TRUNCATE TABLE Employees;
removes all rows and resets the identity automatically.
Considerations and Best Practices
- When to Use Reseeding: Only reset the identity when necessary, as it can affect data consistency.
- Impact on Foreign Keys: If your table has relationships, truncation may not be an option due to foreign key constraints.
- Use in Development vs. Production: Frequent reseeding is more common in development environments for testing scenarios but should be used cautiously in production.
- Avoid Identity Gaps: If you need consecutive numbers without gaps, consider alternative approaches, such as using sequences (
CREATE SEQUENCE
).
Conclusion
Resetting the identity seed in SQL Server is useful when you need a fresh start for numbering records after deletion. Using DBCC CHECKIDENT
or TRUNCATE TABLE
provides different approaches depending on whether you want to retain data integrity or completely reset the table. Understanding these methods helps maintain control over auto-incrementing values in your database, ensuring expected behavior in your applications.
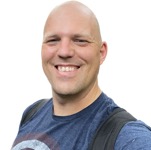
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.